Class is a user-defined data type. It is a collections of data members(variables) and member functions(methods/functions). Class is a blueprint for creating an object.
Syntax of Class
class ClassName {
access_specifier:
// Data members
// Member functions
};
Example of class:
#include<iostream>
using namespace std;
class teacher
{
public:
int id;
string name;
void display(){
cout<<id;
cout<<name;
}
};
int main()
{
teacher r;
r.id=1;
r.name=”rajesh”;
r.display();
return 0;
}
In C++, an object is an instance of a class. You can access public members of class using an object with dot operator. You can create multiple objects from the same class.
Syntax of an object:
ClassName objectName;
Program:
#include <iostream>
using namespace std;
// Class Definition
class Car {
public:
string brand;
int year;
// Function to display car details
void displayDetails() {
cout << “Brand: ” << brand << endl;
cout << “Year: ” << year << endl;
}
};
int main() {
// Creating an object of class Car
Car myCar;
// Assigning values to the object
myCar.brand = “Toyota”;
myCar.year = 2020;
// Calling the member function using the object
myCar.displayDetails();
return 0;
}
2.1 Concept of Object and Class
A class is a user-defined data type that serves as a blueprint for creating an objects.
Class is a collections of data members (variables) and member functions (methods) that operate on the data.
Class Syntax:
Class Syntax:
class ClassName {
public:
// data members
int data;
// member functions
void display();
};
Example Program of Class:
An object is an instance of a class.
When a class is defined, no memory is allocated until an object of that class is created.
Object Syntax:
ClassName obj; // ‘obj’ is an object of ‘ClassName’
2.2 Define Data Member and Member Function
Data Member:
Example:
class Student {
public:
int id;
string name;
};
Member Function: Functions that operate on the data members of a class. Member functions define the behavior of the objects.
Example:
class Student {
public:
int id;
string name;
void display() {
cout << “ID: ” << id << “, Name: ” << name << endl;
}
};
2.3 Create Object and Access Member Function
To create an object and access member functions:
First, define the class.
Create an object of the class.
Use the dot operator . to access the member functions and data members.
Example:
#include <iostream>
using namespace std;
class Student {
public:
int id;
string name;
void display() {
cout << “ID: ” << id << “, Name: ” << name << endl;
}
};
int main() {
Student s1; // Create an object of Student
s1.id = 101; // Access data members
s1.name = “John”;
s1.display(); // Call member function
return 0;
}
2.4 Making Outer Function Inline
In C++, we can declare a function as inline. This copies the function to the location of the function call in compile-time and may make the program execution faster.
An inline function is a function defined with the inline keyword and is meant to be expanded in line when it is invoked. The primary advantage of inline functions is that they can reduce the overhead of function calls by embedding the function code directly at the point of invocation, potentially improving performance for small, frequently called functions.
The compiler may not implement inlining in situations like these:
- If a function contains a loop. (for, while, do-while)
- if a function has static variables.
- Whether a function recurses.
- If the return statement is absent from the function body and the return type of the function is not void.
- Whether a function uses a goto or switch statement.
Syntax:
inline return_type function_name(parameters)
{
// function code?
}
#include <iostream>
using namespace std;
inline int add(int a, int b)
{
return(a+b);
}
int main()
{
cout<<“Addition of ‘a’ and ‘b’ is:”<<add(2,3);
return 0;
}
Example2:
#include <iostream>
using namespace std;
inline void displayNum(int num) {
cout << num << endl;
}
int main() {
// first function call
displayNum(5);
// second function call
displayNum(8);
// third function call
displayNum(666);
return 0;
}
We then called the function 3 times in the main() function with different arguments. Each time displayNum() is called, the compiler copies the code of the function to that call location.
Example 3:
constructor
#include <iostream>
using namespace std;
class MyClass { // The class
public: // Access specifier
int x;
MyClass() {
x=5;// Constructor
cout<<x;
}
};
int main() {
MyClass myObj; // Create an object of MyClass (this will call the constructor)
return 0;
}
Parameterized constructor
Example 1
#include <iostream>
using namespace std;
class SumCalculator {
public:
int number1;
int number2;
// Parameterized constructor with different parameter names
SumCalculator(int n1, int n2) {
number1 = n1;
number2 = n2;
}
// Method to display the numbers and their sum
void displaySum() {
int sum = number1 + number2;
cout << “Number 1: ” << number1;
cout << “\n Number 2:” << number2;
cout << “\n Sum: ” << sum;
}
};
int main() {
// Creating an object using the parameterized constructor
SumCalculator calculator(10, 20);
// Displaying the numbers and their sum
calculator.displaySum();
return 0;
}
Example 2
#include <iostream>
using namespace std;
class Person {
public:
string name;
int age;
// Parameterized constructor with different parameter names
Person(string n, int a) {
name = n;
age = a;
}
// Method to display person’s details
void displayDetails() {
cout << “Name: ” << name << endl;
cout << “Age: ” << age << endl;
}
};
int main() {
// Creating an object using the parameterized constructor
Person person1(“rajesh”, 27);
// Displaying the details of the person
person1.displayDetails();
return 0;
}
Copy Constructor
Example 1
//copy constructor
#include<iostream>
using namespace std;
class raju{
public:
int x;
int y;
raju(int a){
x=a;
}
raju(raju &ref){
y=ref.x;
cout<<“copy first constructor instance value: “<<y<<endl;
}
};
int main(){
raju r(20);
raju r1(r);
return 0;
}
Example 2
#include<iostream>
using namespace std;
class copycons{
int x;
int y;
public:
copycons(int a, int b){
x=a;
y=b;
}
copycons(copycons &ref){
x=ref.x;
y=ref.y;
}
void display()
{
cout<<“\n x is: “<<x;
cout<<“\n y is: “<<y;
}
};
int main()
{
copycons obj(10,20);
copycons obj2(obj);
obj.display();
obj2.display();
return 0;
}
Destructor
A destructor is a special member function of a class that is executed when an object of that class is destroyed. It is used to release resources that the object may have acquired during creation. The destructor has the same name as the class, prefixed with a tilde (~), and it cannot take any parameters nor return any value. It can be defined only one in class. A destructor works opposite to constructor.
Syntax:
class ClassName {
public:
~ClassName() {
// Destructor body
}
};
Example 1
#include <iostream>
using namespace std;
class rajeshtuts
{
public:
rajeshtuts()
{
cout<<“Constructor Invoked when object is created”<<endl;
}
~rajeshtuts()
{
cout<<“Destructor Invoked when object is instantiated”<<endl;
}
};
int main(void)
{
rajeshtuts e1; //creating an object of Employee
return 0;
}
Example 2
// C++ program to demonstrate the number of times
// constructor and destructors are called
#include <iostream>
using namespace std;
// It is static so that every class object has the same
// value
static int Count = 0;
class Test {
public:
// User-Defined Constructor
Test()
{
// Number of times constructor is called
Count++;
cout << “No. of Object created: ” << Count << endl;
}
// User-Defined Destructor
~Test()
{
// It will print count in decending order
cout << “No. of Object destroyed: ” << Count
<< endl;
Count–;
// Number of times destructor is called
}
};
// driver code
int main()
{
Test t, t1, t2, t3;
return 0;
}
Friend Function
Friend Function: A friend function can access the private and protected data of a class. We declare a friend function using the friend keyword inside the body of the class.
For accessing the data, the declaration of a friend function should be done inside the body of a class starting with the keyword friend. The function definition does not use either the keyword friend or scope resolution operator.
Syntax of Friend function:
class class_name
{
friend data_type function_name(argument/s);
};
Example2:
#include<iostream>
using namespace std;
class ffaddition{
private:
int x;
int y;
public:
void get_data();
friend int sum(ffaddition);
};
void ffaddition::get_data(){
cout<<“enter two numbers:”;
cin>>x>>y;
}
int sum(ffaddition ref){
return(ref.x+ref.y);
}
int main(){
ffaddition ff;
ff.get_data();
cout<<“sum is: “<<sum(ff);
return 0;
}
Hierarchical Inheritance Example
#include<iostream>
using namespace std;
class A{
public:
void a(){
cout<<“class a function called\n”;
}
};
class B:public A{
public:
void b(){
cout<<“class b function called\n”;
}
};
class C:public A{
public:
void c(){
cout<<“class C function called\n”;
}
};
int main()
{
B obj1;
obj1.b();
obj1.a();
C obj2;
obj2.c();
obj2.a();
return 0;
}
C++ program to demonstrate the template function(Generic Function)
#include <iostream>
using namespace std;
// Template function for addition
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
int intResult = add(5, 10); // Adding two integers
double doubleResult = add(3.14, 2.71); // Adding two doubles
float floatResult = add(1.5f, 2.5f); // Adding two floats
cout << “Sum of 5 and 10 is: ” << intResult << endl; // Output: 15
cout << “Sum of 3.14 and 2.71 is: ” << doubleResult << endl; // Output: 5.85
cout << “Sum of 1.5 and 2.5 is: ” << floatResult << endl; // Output: 4
return 0;
}
#include <iostream>
using namespace std;
// Template function to print data
template <typename T>
void printData(T data) {
cout << “Data: ” << data << endl;
}
int main() {
int intValue = 100;
double doubleValue = 99.99;
char charValue = ‘A’;
string stringValue = “Hello, World!”;
printData(intValue); // Output: Data: 100
printData(doubleValue); // Output: Data: 99.99
printData(charValue); // Output: Data: A
printData(stringValue); // Output: Data: Hello, World!
return 0;
}
C++ program to demonstrate the template class
#include <iostream>
template <typename T>
class MyClass {
public:
T data;
// Member function to display the data
void display() {
std::cout << “Data: ” << data << std::endl;
}
};
int main() {
MyClass<int> intObj; // Instance of MyClass with int type
intObj.data = 42; // Assign value to data
intObj.display(); // Output: Data: 42
MyClass<double> doubleObj; // Instance of MyClass with double type
doubleObj.data = 3.14; // Assign value to data
doubleObj.display(); // Output: Data: 3.14
return 0;
}
File Handling in C++
In C++, file handling is done using the standard library’s <fstream> header, which provides classes to handle input and output file streams. There are three main classes for file handling:
ifstream: For reading from files (input stream).
ofstream: For writing to files (output stream).
fstream: For both reading and writing (input/output stream).
File Operations
- Read
- Write
- open
- Close
- End of file
- Update
File Modes
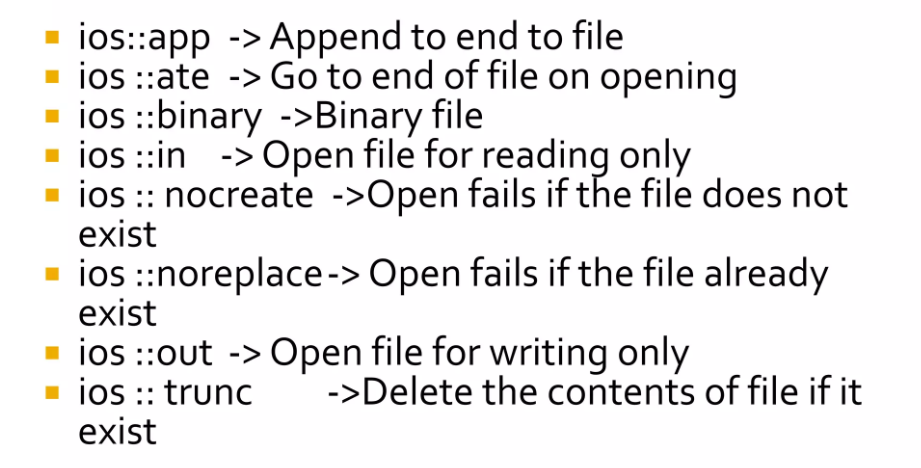
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ofstream file(“example.txt”); // Open file for writing
if (file.is_open()) {
file << “Writing to the file.\n”;
file.close(); // Always close the file
} else {
cout << “Unable to open file.”;
}
return 0;
}
Exam Writing Tips:
- Use / make Graph or picture to clear the concepts,
- Prefer to write in points not in paragraph,
- Write definition and make simple program on that particular questions with logic
- If you cannot write program, write algorithm or syntax using structure of C++ program.
Important Topics
Define C++ and Features/characteristics of C++/Opps
What is object oriented Programming Language
- Class – Meaning and represent simple program using class
- Objects- Meaning and represent simple program using objects
- Encapsulation- Meaning and represent simple program using Encapsulation
- Abstraction- Meaning and represent simple program using abstraction
- Polymorphism Meaning and represent simple program using polymorphism
- Inheritance- Meaning and represent simple program using inheritence
Data types in C++
difference between array and pointer
Meaning of dynamic memory and Dynamic memory allocation methods in C++
Difference between Call by value and call by reference
Function Overloading
Write a program to solve divide by zero exception handling by using try, throw and catch keywords.
Define template and its types with simple program example
Advantages of template
C++ (OOPS)
* Concept of Template
Template is a frame or blueprint which allow us to create generic classes and generic functions. The keyword template is used to define function template and class template. In C++, Using Template keyword, we can work with different datatype with same functions and classes with same arguments and parameters, no need to write same code again and again for declaring different data type. C++ allows generic classes and functions to handle different data types.
Template can be represented in two ways:
Function template: Function Template is used to define a function to handle arguments of different types in different times.
Example of Function Template
Write C++ program to find greatest number from two numbers by using template Function.
#include<iostream.h>
#include<conio.h>
using namespace std;
template <class X>
x big(x a, x b)
{
if(a>b)
return(a);
else
return(b);
}
int main()
{
cout<<big(4,5);
cout<<big(5.8,3.5);
getch();
}
*Function Overloading Templates
Example of Overloading Function Template
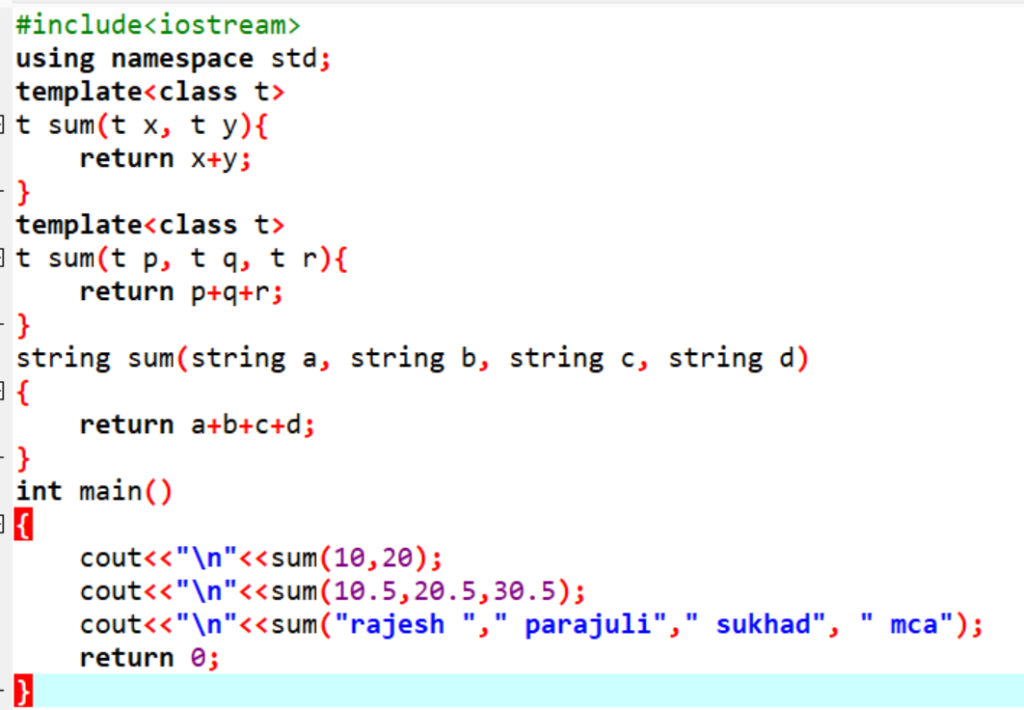
Example 2:
#include<iostream.h>
#include<conio.h>
using namespace std;
template <class T>
T Max(T a, T b)
{
if(a>b)
return a;
else
return b;
}
template <class T>
T Max(T a, T b, Tc)
{
if(a>b && a>c)
return a;
else if(b>a && b>c)
return b;
else
return c;
}
void main()
{
int a,b,c;
cout<<“Enter three numbers:”;
cin>>a>>b>>c;
cout<<“Maximum number among “<<a<<” and “<<b<<” is: “<<Max(a,b)<<end1;
cout<<“Maximum number among “<<a<<” and “<<b<<” and <<c<<” is: “<<Max(a,b,c)<<end1;
getch();
}
output:
Enter three numbers:1 4 65
Maximum number among 1 and 4 is:4
Maximum number among 1, 4 and 65 is:65
Paradigm can also be termed as method to solve some problem or do some task. Programming paradigm is an approach to solve problem using some programming language or also we can say it is a method to solve a problem using tools and techniques that are available to us following some approach. There are lots for programming language that are known but all of them need to follow some strategy when they are implemented and this methodology/strategy is paradigms. Apart from varieties of programming language there are lots of paradigms to fulfill each and every demand. They are discussed below:
1. Imperative programming paradigm: It is one of the oldest programming paradigm. It features close relation to machine architecture. It is based on Von Neumann architecture. It works by changing the program state through assignment statements. It performs step by step task by changing state. The main focus is on how to achieve the goal. The paradigm consist of several statements and after execution of all the result is stored
Advantages:
- Very simple to implement
- It contains loops, variables etc.
Disadvantage:
- Complex problem cannot be solved
- Less efficient and less productive
- Parallel programming is not possible
Imperative programming is divided into three broad categories: Procedural, OOP and parallel processing. These paradigms are as follows:
- Procedural programming paradigm –
This paradigm emphasizes on procedure in terms of under lying machine model. There is no difference in between procedural and imperative approach. It has the ability to reuse the code and it was boon at that time when it was in use because of its reusability.
- Object oriented programming –
The program is written as a collection of classes and object which are meant for communication. The smallest and basic entity is object and all kind of computation is performed on the objects only. More emphasis is on data rather procedure. It can handle almost all kind of real life problems which are today in scenario.
Advantages:
- Data security
- Inheritance
- Code reusability
- Flexible and abstraction is also present
Object-oriented analysis and design (OOAD) is a technical approach for analyzing and designing an application, system, or business by applying object-oriented programming, as well as using visual modeling throughout the software development process to guide stakeholder communication and product quality.