Web Technology
Assignments
Study Materials
- Unit 1 Introduction to HTML
- Unit 2 Cascading Style Sheet
- Unit 3
- Unit 4
- Unit 5
- Unit 6
Old Exam Questions answers Chapterwise
- Unit 1 Introduction to HTML
- Unit 2 Cascading Style Sheet
- Unit 3 Client side JavaScript program
- Unit 4 Server Side Program PHP
- Unit 5 Working with Web Forms
- Unit 6 Database and PHP
HTML(Hypertext Markup Language) is a standard language that helps us to build web pages on the internet. Html contains a series of tag elements that can be used as a way to tell your web browsers about the structure of your web page, like where the headings are, where the images are, and so on. Some browsers does not support some html tag elements.
To build a website, you need to follow these steps:
- Create a html file using any text editor (example: NOTEPAD, SUBLINE, VISUAL STUDIO, VIM ETC.)
- Add all the details that you want to see on your website in that html file.
- Save the file as file_name.html extension.
- Right click on the file where you saved and go to open with option and select any browser (e.g. Google chrome, Firefox, Opera, Safari) to open the file.
Structure / Skeleton of HTML
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<title> page title</title>
<meta name=”viewport” content=”width=device-width, initial-scale=1″>
<link rel=”stylesheet” href=”externalcss”>
<style>
internal css
</style>
<script type=”text/javascript” src=”script.js”></script>
</head>
<body>
<img src=”url of image” alt=”” title=”” width=”200px” height=”500px”>
<h1> This is a heading</h1>
<p> This is a paragraph</p>
<p> This is another paragraph.</p>
<script type=”text/javascript”>
another way
</script>
</body>
</html>
Block and Inline Elements
Html contains two types of elements: Inline and Block. A web page is developed by the combination of Inline and Block elements.
Inline Elements: Inline elements are those elements that do not start on a new line when you insert the element in the code. Using Inline elements, you can add space to the left or right side of an inline element but you cannot add space on the top and bottom of the element. Some Inline Elements are <span>, <a>, <img>, <strong>, <sub>, <sup>, <time>, <label> ..etc.
<html>
<body>
<p> The span tag is an <span> inline element.</span> Another inline element is label also.</p>
</body>
</html>
Output:
The span tag is an inline element. Another inline element is label also.
Block Elements: Block elements are working as just oppositive of Inline elements. Block elements start on a new line and they take full width of the webpage for the content. You can add top and bottom margins to these elements and they also create a larger structure than inline elements. Some Block Elements are <div>, <p>, <li>, <form>, <video>,<h1> to <h6>, <table> …etc.
<html>
<body>
<p> Hello web developers.</p>
<li> you need to know html</li>
<li> you need to know css</li>
<li> you need to know javascript</li>
<li> you need to know php</li>
</body>
</html>
Output: Hello web developers.
- you need to know html
- you need to know css
- you need to know javascript
- you need to know php
Difference Between Block Elements and Inline Elements
Block Elements | Inline Elements |
---|---|
|
|
|
|
|
|
|
|
|
|
|
|
Examples of Block Elements are :
| Examples of Inline Elements :
|
Demonstrate Text Formatting in HTML
HTML contains several elements for defining text with a special meaning. Formatting elements are used to display special types of text.
<b> – Bold text
<strong> – Important text
<i> – Italic text
<em> – Emphasized text
<mark> – Marked text
<small> – Smaller text
<del> – Deleted text
<ins> – Inserted text
<sub> – Subscript text
<sup> – Superscript text
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<title> page title</title>
<meta name=”viewport” content=”width=device-width, initial-scale=1″>
</head>
<body>
<b>this text is bold</b>
<strong> this text is important and displayed in bold</strong>
<i> this is italic</i>
<em> this text is emphasized and displayed like italic</em>
<small> this is smaller text</small>
<p> this marked something <mark>important </mark> text</p>
<p> this is del used to <del> delete something</del> unneccessary item</p>
<ins> insert text or something you want</ins>
<sub> subscripted text</sub>
<sup> superscripted text</sup>
</body>
</html>
List in HTML
Lists is a collection of items, and one of the best ways of representing information. In HTML, lists are one of the most frequently used elements, whether it’s for navigation or for representing general content.
HTML Lists are used to specify lists of information. All lists may contain one or more list elements. There are three different types of HTML lists:
- Ordered List or Numbered List (ol)
- Unordered List or Bulleted List (ul)
- Description List or Definition List (dl)
HTML Ordered List: It is also known as numbered list. The ordered list starts with <ol> tag and the list items start with <li> tag. In ordered list, we use type attribute to define its type which represent the lists like type=”A”, it lists the items in A list_items, B list_items, etc. By defaults, All the list items are marked with numbers.
Example of ordered list:
<!DOCTYPE html>
<html>
<head>
<title>Book list</title>
</head>
<body>
<h2>An ordered HTML List of Books Name</h2>
<0l type=”A”>
<li>Web Technology</li>
<li>Digital Logics</li>
<li>Math</li>
<li>English</li>
</0l>
</body>
</html>
HTML Unordered List: Unordered list is also known as bulleted list. The Unordered list starts with <ul> tag and list items start with the <li> tag. In unordered list, we use ‘type’ attribute to define its type which represent the lists like type=”square”, it lists the items in square symbol list_item, square symbol list_item, etc. By defaults, All the list items are marked with Bullets.
Example of Unordered list:
<!DOCTYPE html>
<html>
<head>
<title>Book list</title>
</head>
<body>
<h2>An unordered HTML List of Books Name</h2>
<ul type=”disc”>
<li>Web Technology</li>
<li>Digital Logics</li>
<li>Math</li>
<li>English</li>
</ul>
</body>
</html>
HTML Description List or Definition List: It is also known as definition list where entries are listed like a dictionary or encyclopedia. The description list is very useful when you want to present glossary, list of terms or other name-value list.
Example of Definition / description list:
<!DOCTYPE html>
<html>
<body>
<h2>A Description List</h2>
<dl>
<dt>COURSE</dt>
<dd>- Ghodaghodi Multiple Campus has BICTE course. </dd>
<dt>LIBRARY</dt>
<dd>- Ghodaghodi Multiple Campus has LIBRARY Facility.</dd>
<dt>BUS</dt>
<dd>- Ghodaghodi Multiple Campus has bus Facility for students.</dd>
</dl>
</body>
</html>
Multimedia in HTML(Image, audio, video, and youtube player)
Audio
<!DOCTYPE html>
<html>
<body>
<audio controls autoplay unmuted loop>
<source src=”horse.ogg” type=”audio/ogg”>
<source src=”horse.mp3″ type=”audio/mpeg”>
Your browser does not support the audio element.
</audio>
</body>
</html>
Video
<!DOCTYPE html>
<html>
<body>
<video width=”320″ height=”240″ autoplay unmuted loop>
<source src=”movie.mp4″ type=”video/mp4″>
<source src=”movie.ogg” type=”video/ogg”>
Your browser does not support the video tag.
</video>
</body>
</html>
Form Elements (text, password, file, radio, checkbox, textarea, hidden, select option, button, date, email )
<!Doctype Html>
<Html>
<Head>
<Title>
Create a Registration form
</Title>
</Head>
<Body>
The following tags are used in this Html code for creating the Registration form:
<br>
<form>
<label> Firstname </label>
<input type=”text” name=”firstname” size=”15″/> <br> <br>
<label> Lastname: </label>
<input type=”text” name=”lastname” size=”15″/> <br> <br>
<label>
Course :
</label>
<select>
<option value=”Course”>Select Course</option>
<option value=”BICTE”>BICTE</option>
<option value=”BBS”>BBS</option>
<option value=”MBS”>MBS</option>
<option value=”MBA”>MBA</option>
<option value=”MA”>M.A</option>
<option value=”BA”>BA</option>
</select>
<br>
<br>
<label>
Gender :
</label><br>
<input type=”radio” name=”gender”/> Male <br>
<input type=”radio” name=”gender”/> Female <br>
<input type=”radio” name=”gender”/> Other
<br>
<br>
<label>
Hobbies:
</label>
<br>
<input type=”checkbox” name=”Programming”> Programming <br>
<input type=”checkbox” name=”Cricket”> Cricket <br>
<input type=”checkbox” name=”Football”> Football <br>
<input type=”checkbox” name=”reading Novel”> Reading Novel <br>
<br>
<br>
<label>
Phone :
</label>
<input type=”text” name=”phone” size=”10″> <br> <br>
Address
<br>
<textarea cols=”80″ rows=”5″ value=”address”>
</textarea>
<br> <br>
Email:
<input type=”email” id=”email” name=”email”> <br>
<br> <br>
Password:
<input type=”Password” id=”pass” name=”pass”> <br>
<br> <br>
<input type=”submit” value=”Submit”>
<input type=”reset” value=”Reset”>
</form>
</Body>
</Html>
Cascading Stylesheet (CSS)
Selectors are used to select (or target) HTML elements so you can apply styles to them.
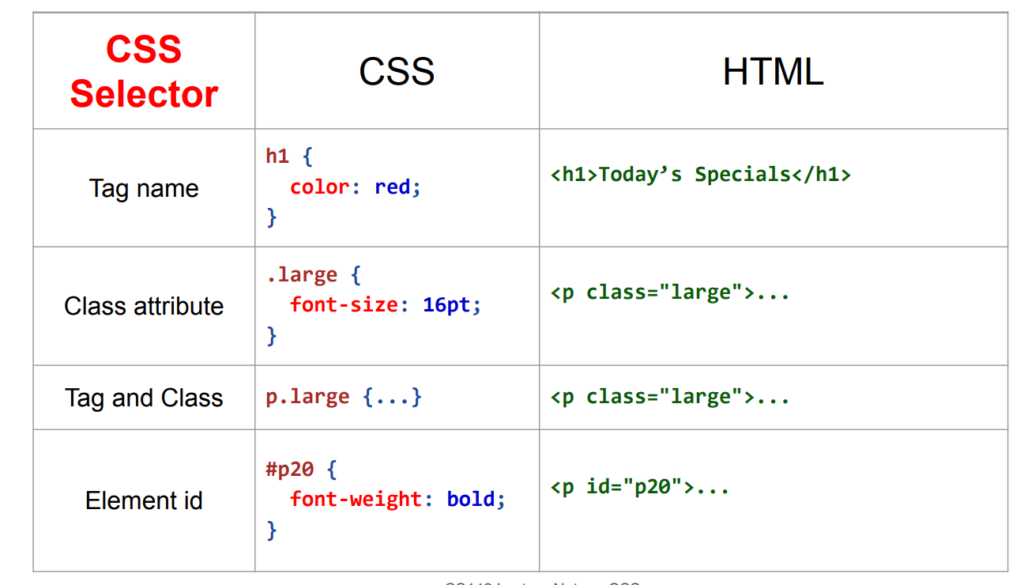