OOP (Object Oriented Programming)
Object Oriented programming is a programming paradigm based on the concepts of objects which can contain data and methods. Many of programming language supports the concepts of object oriented Programming. C++, Java, Python are the example programming language of OOP.
Features of Object Oriented Programming are:
- Class
- Object
- Polymorphism
- Inheritance
- Encapsulation
- Abstraction
1. Class: Class is a user-defined data type. Class is a blueprint for creating an objects. Class contains data members and member functions.
Syntax:
class className
{
access specifier:
data_type data_members;
data_type member_functions;
};
Class Example:
class student
{
public:
int rollno;
string name;
void show();
};
2.Objects: Object is an instance of a class.
Syntax:
className objectName;
Example:
student st;
3.Polymorphism: Polymorphism means “many forms.” It allows methods to perform differently based on the object that calls them. Example: Function Overloading, Function Overriding
Types of Polymorphism:
- Compile-time Polymorphism
- Runtime Polymorphism
4.Inheritance: The process of creating new class derived class by acquiring the properties of base class is known as inheritance. Inheritance allows a new class (derived class / child class) to acquire the properties and methods of an existing class (base class / parent class / super class).
Types of Inheritance:
- Single Inheritance
- Multi-level Inheritance
- Multiple Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Single Inheritance: A Derived class inherits the properties and methods of one base class.
Syntax:
class A
{
access specifier:
data_type data_members of A;
data_type member_functions of A;
};
class B: public A
{
access specifier:
data_type data_members of B;
data_type member_functions of B;
};
Multilevel Inheritance: A process of deriving a class from another derived class.
Syntax:
class A
{
data_members of class A;
member_functions of class A;
};
class B: access specifier A
{
Data_members of class B;
member_functions of class B;
};
Example:
class A
{
public:
int A_id;
string A_name;
void show();
};
class B: public A
{
public:
int B_id;
string B_name;
void display();
};
Multiple Inheritance: A derived class inherits from more than one base classes. This allows the derived class to have access to members of multiple base classes.
Syntax:
class A
{
data_members of class A;
member_functions of class A;
};
class B: {
Data_members of class B;
member_functions of class B;
};
class C: accessSpecifier A, accessSpecifier B{
Data_members of class B;
member_functions of class B;
};
Example:
class A
{
public:
int A_id;
string A_name;
void show();
};
class B
{
public:
int B_id;
string B_name;
void display();
};
class C: public A, public B
{
public:
int B_id;
string B_name;
void display();
};
Hierarchical Inheritance: Hierarchical Inheritance is a type of inheritance where multiple derived classes inherit from a single base class. It has tree like structure.
Syntax:
class A
{
data_members of class A;
member_functions of class A;
};
class B: accessSpecifier A
{
Data_members of class B;
member_functions of class B;
};
class C: accessSpecifier A
{
Data_members of class C;
member_functions of class C;
};
Example:
class A
{
public:
int A_id;
string A_name;
void show();
};
class B: public A
{
public:
int B_id;
string B_name;
void display();
};
class C: public A
{
public:
int B_id;
string B_name;
void display();
};
5.Encapsulation: Encapsulation in C++ means binding the data (variables) and the functions (methods) that work on the data together in a single unit. Class is the best example of encapsulation.
6.Abstraction: Abstraction in C++ is a fundamental concept in Object-Oriented Programming (OOP) that focuses on hiding implementation details and exposing only the essential features of an object.
Advantages of Object Oriented Programming
- Reusability: Code can be reused through inheritance, saving time and effort.
- Modularity: Programs are divided into smaller, manageable parts called objects.
- Flexibility: You can use polymorphism to write flexible and easily extendable code.
- Data Security: Encapsulation protects data by restricting direct access.
- Scalability: OOP makes it easy to add new features without affecting existing code.
- Real-world Mapping: It models programs based on real-world entities, making them intuitive.
- Improved Productivity: Clear structure and reusable code make development faster.
- Easy Maintenance: Code is organized and modular, making debugging and updates simpler.
Disadvantages of OOP Object Oriented Programming
- Complexity: Designing and understanding object relationships can be challenging.
- Performance Overhead: OOP uses more memory and processing power compared to procedural programming.
- Not Suitable for All Problems: Simple tasks may become unnecessarily complex with OOP.
- Learning Steep Curve: Concepts like inheritance, polymorphism, and encapsulation can be difficult for beginners.
- Code Duplication for Simple Projects: OOP might lead to more boilerplate code in small programs.
- Maintenance Issues with Large Projects: If not designed well, managing object interactions in big systems can become problematic.
- Overhead in Debugging: Tracking bugs across multiple objects and classes can be time-consuming.
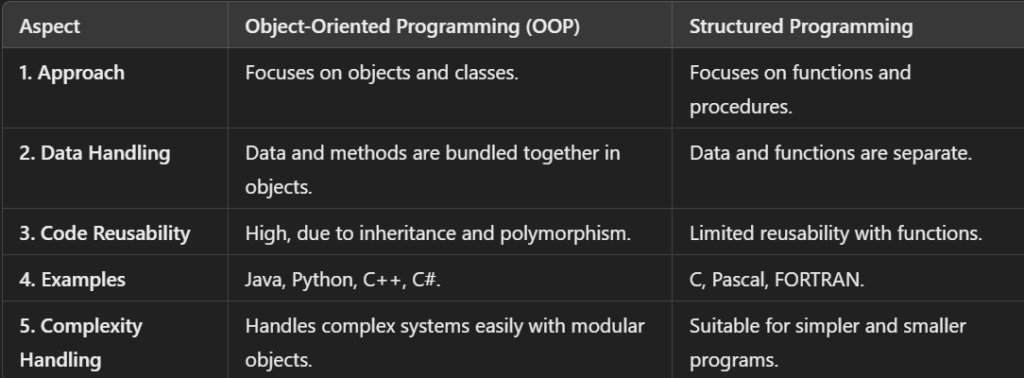
Exercises (Exam Oriented)

Software project management
A software project is the step-by-step process of creating a software in a well defined, systematic and scientific manner. It starts with understanding the user needs (requirement gathering) and includes designing, building, testing, and maintaining the software, carried out according to the execution methodologies in a specified period of time to achieve intended software product.